As you might know, the WordPress Block Editor (aka Gutenberg) makes use of Block Categories to structure the various registered blocks. This can be seen, for example, in the Block Picker:
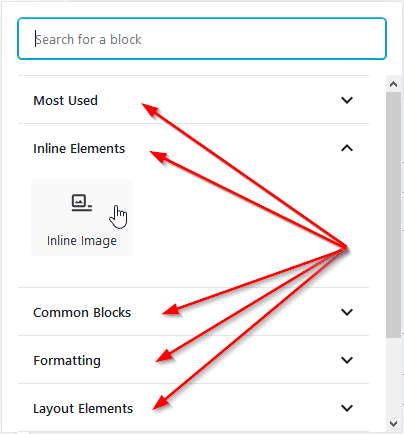
Now, what we often want or have to do is to register a custom block category. And that’s possible.
The official way to do so is by using the block_categories
filter, that is, in the PHP part of your code base:
add_filter( 'block_categories', function ( $categories ) {
$categories[] = [
'slug' => 'tf-blocks',
'title' => __( 'TF Blocks', 'tf_blocks' ),
];
return $categories;
} );
That’s OK, and it works.
However, sometimes you don’t really need the category anywhere else in your PHP code, but in JavaScript. That is when you (mostly or only) register custom blocks in JavaScript, and not from within your PHP code.
Now, to use the exact same category (reference) in JS, you could either just hard-code it (several times), which is not a good idea, in general. Or you could export the data to JavaScript (i.e., use wp_localize_script()
).
But wouldn’t it be nice if you could also actually register a block category in JavaScript? I do think so.
Unfortunately, there is no dedicated function to add or register a single block category. So I leveraged what Core gave me, and turned this into a simple function:
const { dispatch, select } = wp.data;
/**
* Register the given block category.
*
* @param {string} slug - Category slug.
* @param {string} title - Category title.
* @param {Function|string} [icon=null] - Optional. Category icon. Defaults to null.
*/
export function registerBlockCategory( slug, title, icon = null ) {
const { getCategories } = select( 'core/blocks' );
const { setCategories } = dispatch( 'core/blocks' );
setCategories( [
...( getCategories() || [] ),
{
icon,
slug,
title,
},
] );
}
Now, you can call this function to register a custom block. No PHP required.
Maybe that’s helpful for anyone, although it’s no Black Magic.
Oh, and by the way, make sure to register any custom category before registering a block for that very category. Gutenberg will just die.
Leave a Reply